How to Use Checkbox in Ruby on Rails
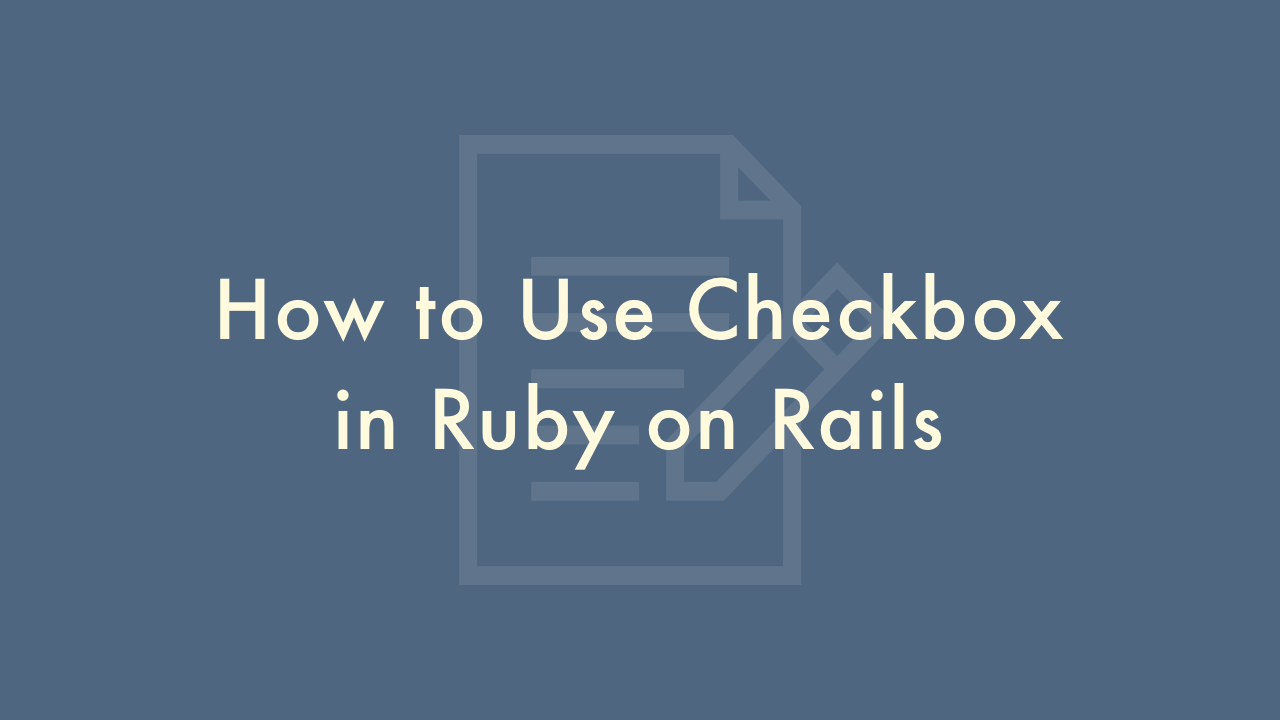
Contents
In this article, you will learn how to use checkbox in Ruby on Rails.
Using the check_box form helper method
In Ruby on Rails, you can use the check_box form helper method to create a checkbox input field in a form. Here is an example:
<%= form_for @user do |f| %>
<%= f.label :is_admin, "Admin" %>
<%= f.check_box :is_admin %>
<%= f.submit "Submit" %>
<% end %>
In the example above, @user is an instance variable representing the user model, and :is_admin is a boolean attribute of the user model. The f.check_box :is_admin creates a checkbox input field for the is_admin attribute.
When the form is submitted, the checkbox value will be submitted as either “1” (checked) or “0” (unchecked). You can use this value to update the is_admin attribute of the user model.
To display the current value of the checkbox in the form, you can set the checked option of the check_box method to the current value of the attribute. For example:
<%= f.check_box :is_admin, checked: @user.is_admin %>
This will check the checkbox if the is_admin attribute of the @user object is true, and leave it unchecked if the attribute is false.
Using the check_box_tag helper method
<%= form_tag do %>
<%= label_tag :is_admin, "Admin" %>
<%= check_box_tag :is_admin %>
<%= submit_tag "Submit" %>
<% end %>
In this example, form_tag is a helper method for creating a form without a model object, and check_box_tag is a helper method for creating a checkbox input field without a model attribute. check_box_tag takes the name of the input field as its first argument, and the value to be submitted when the checkbox is checked as its second argument. If the checkbox is unchecked, no value will be submitted.
Using the fields_for method with a nested model
<%= form_for @user do |f| %>
<%= f.fields_for :permissions do |permissions_fields| %>
<%= permissions_fields.label :can_edit, "Edit" %>
<%= permissions_fields.check_box :can_edit %>
<% end %>
<%= f.submit "Submit" %>
<% end %>
In this example, @user is a model object with a nested permissions model. fields_for is a helper method for creating fields for a nested model. permissions_fields is a variable representing the fields for the permissions model. permissions_fields.check_box :can_edit creates a checkbox input field for the can_edit attribute of the permissions model.