How to Use the Ruby Time Class
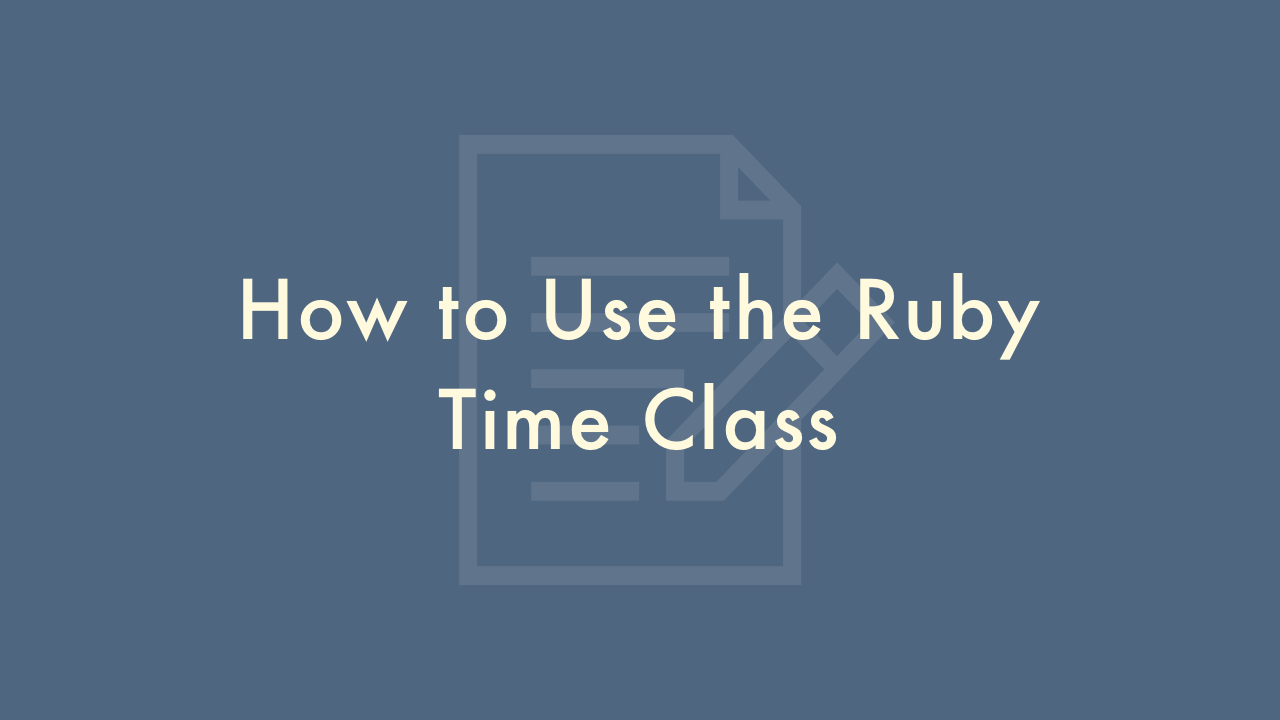
Contents
In this article, you will learn how to use the Ruby Time class.
Using the Ruby Time Class
The Ruby Time class is a built-in class in Ruby that represents a specific moment in time. It provides a range of methods for working with dates and times.
To use the Time class, you first need to require it in your program using:
require 'time'
Creating a Time Object
To create a new Time object, you can use one of the following methods:
-
Time.new: creates a Time object representing the current time
Time.new
-
Time.local(year, month, day, hour, min, sec): creates a Time object representing a specific local time
Time.local(2023, 3, 9, 10, 30, 0)
-
Time.utc(year, month, day, hour, min, sec): creates a Time object representing a specific UTC time
Time.utc(2023, 3, 9, 10, 30, 0)
Retrieving Information from a Time Object
Once you have a Time object, you can retrieve various pieces of information from it, such as the year, month, day, hour, minute, second, and timezone.
time = Time.new # create a Time object representing the current time
puts time.year # prints the current year
puts time.month # prints the current month
puts time.day # prints the current day of the month
puts time.hour # prints the current hour
puts time.min # prints the current minute
puts time.sec # prints the current second
puts time.zone # prints the current timezone
You can also compare Time objects to each other using the comparison operators (<, <=, ==, >=, >).
time1 = Time.local(2023, 3, 9, 10, 30, 0)
time2 = Time.utc(2023, 3, 9, 10, 30, 0)
puts time1 == time2 # prints false
puts time1 < time2 # prints true
puts time1 > time2 # prints false
Formatting a Time Object
You can format a Time object using the strftime method. This method takes a string argument that specifies the format of the output. Some common format codes are:
- %Y: year
- %m: month (01..12)
- %d: day of the month (01..31)
- %H: hour of the day, 24-hour clock (00..23)
- %M: minute of the hour (00..59)
- %S: second of the minute (00..60)
- %z: time zone as hour and minute offset from UTC (+HHMM or -HHMM)
time = Time.new
puts time.strftime("%Y-%m-%d %H:%M:%S %z") # prints something like "2023-03-09 10:30:00 +0900"
Converting a Time Object to Other Formats
You can convert a Time object to other formats using the following methods:
-
to_i: returns the Unix timestamp (the number of seconds since January 1, 1970 UTC)
time = Time.new puts time.to_i # prints the current Unix timestamp
-
to_f: returns the Unix timestamp as a floating-point number
time = Time.new puts time.to_f # prints the current Unix timestamp as a floating-point number
-
to_s: returns the time in a human-readable format
time = Time.new puts time.to_s # prints something like "2023-03