How to Resize Images in Python
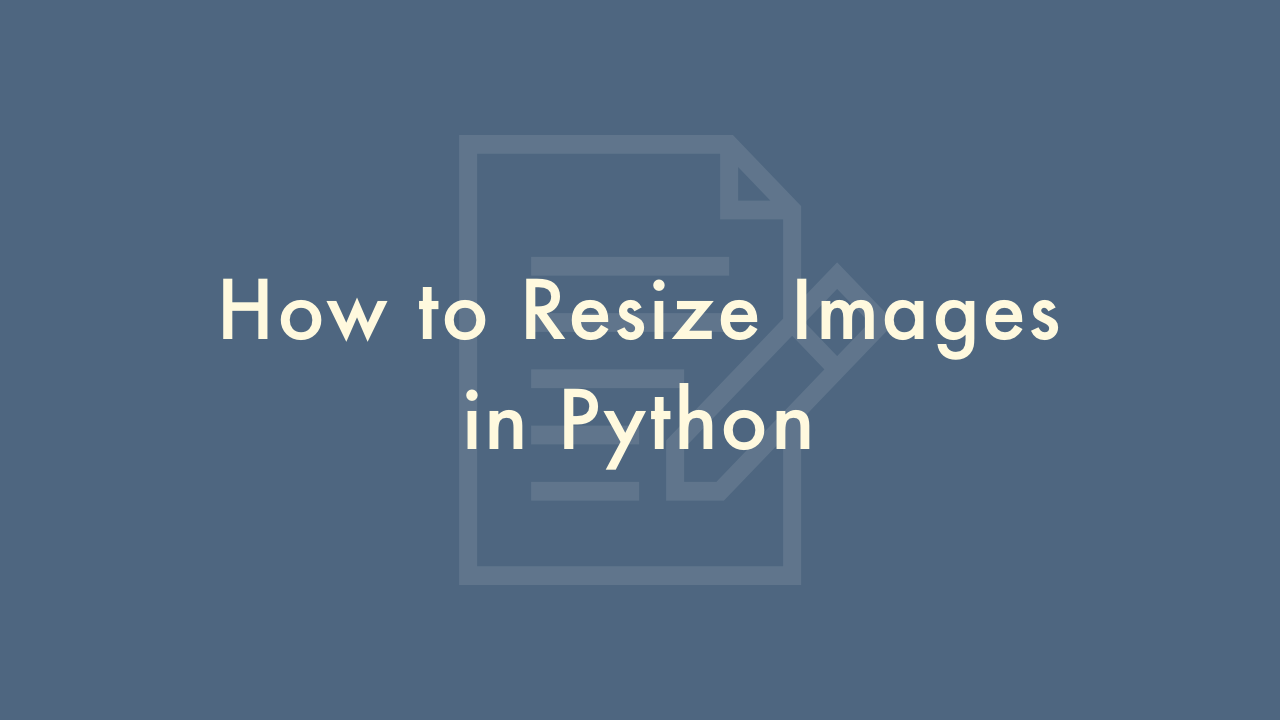
Contents
In this article, you will learn how to resize images in Python.
How to resize images
There are several ways to resize images in Python, but one of the most popular libraries for image processing is the Pillow library. Here’s an example of how to use Pillow to resize an image:
First, you need to install the Pillow library using pip:
pip install Pillow
Once you have installed Pillow, you can use the following code to resize an image:
from PIL import Image
# Open the image file
image = Image.open("image.jpg")
# Set the new size
new_size = (500, 500)
# Resize the image
resized_image = image.resize(new_size)
# Save the resized image
resized_image.save("resized_image.jpg")
In this example, we open an image file using Image.open() from the Pillow library. We then set the new size of the image to be 500×500 pixels using a tuple (500, 500). We then use the resize() function to resize the image and save the resized image using save() function.
You can also choose to preserve the aspect ratio of the image by setting only one of the dimensions (width or height) and letting the other be calculated automatically. For example, if you want to resize the image to be 500 pixels wide and let the height be calculated automatically, you can use the following code:
from PIL import Image
# Open the image file
image = Image.open("image.jpg")
# Set the new width
new_width = 500
# Calculate the new height to preserve the aspect ratio
width, height = image.size
aspect_ratio = height / width
new_height = int(new_width * aspect_ratio)
# Resize the image
new_size = (new_width, new_height)
resized_image = image.resize(new_size)
# Save the resized image
resized_image.save("resized_image.jpg")
In this example, we calculate the new height of the image by preserving the aspect ratio of the original image. We then resize the image and save it using the same code as before.