How to Use the Python Numpy dot() Function
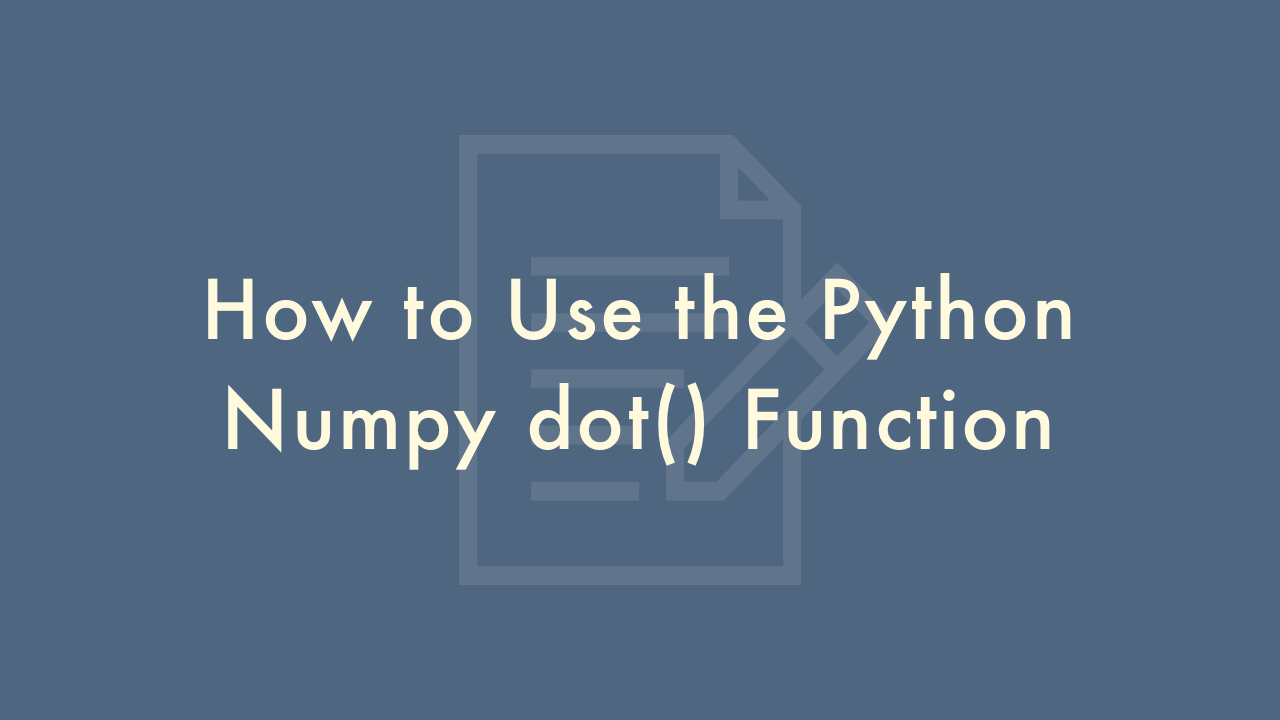
09/13/2021
Contents
In this article, you will learn how to use the Python numpy dot() function.
Python Numpy dot() Function
The NumPy dot() function is used to perform matrix multiplication. It can also be used to perform dot products of vectors, and for multiplying a vector by a matrix.
Syntax
Here’s the syntax of the dot() function:
numpy.dot(a, b, out=None)
Parameters
a
,b
: The input matrices or arrays to be multipliedout
: An optional parameter for the output matrix. If the out parameter is not specified, the result is returned as a new array.
Example
Here are some examples of how to use the dot() function:
Multiplying two matrices
import numpy as np
# Define two matrices
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
# Perform matrix multiplication
C = np.dot(A, B)
print(C)
# Output:
array([[19, 22],
[43, 50]])
Dot product of two vectors
import numpy as np
# Define two vectors
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# Compute dot product
c = np.dot(a, b)
print(c)
# Output: 32
Multiplying a matrix by a vector
import numpy as np
# Define a matrix and a vector
A = np.array([[1, 2], [3, 4]])
b = np.array([5, 6])
# Multiply the matrix by the vector
c = np.dot(A, b)
print(c)
# Output: array([17, 39])
In all the examples above, the dot() function was used to perform matrix multiplication. The first argument is the left matrix, the second argument is the right matrix, and the output is the result of the multiplication.