How to Get All Values from an Associative Array in JavaScript
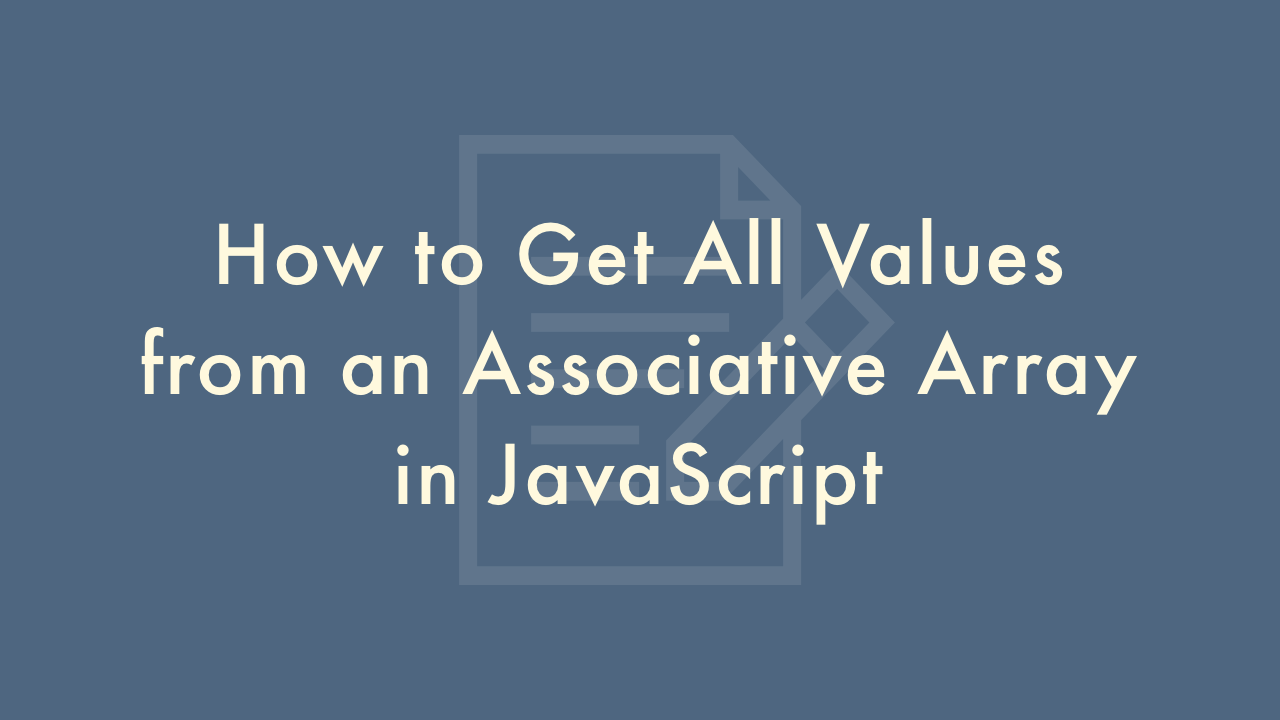
Contents
In this article, you will learn how to get all values from an associative array in JavaScript.
Getting all values from an associative array in JavaScript
In JavaScript, associative arrays are implemented using objects. Here are some methods you can use to get all values from an associative array:
Using the Object.values() method
This method returns an array of all values of an object. You can use this method to get all values from an associative array.
Example
const obj = { a: 1, b: 2, c: 3 };
const values = Object.values(obj);
console.log(values); // Output: [1, 2, 3]
Using a for…in loop
This loop allows you to iterate over the properties of an object, including its values. You can use this loop to get all values from an associative array.
Example
const obj = { a: 1, b: 2, c: 3 };
const values = [];
for (const key in obj) {
values.push(obj[key]);
}
console.log(values); // Output: [1, 2, 3]
Using the Object.entries() method
This method returns an array of all key-value pairs of an object as an array of arrays. You can use this method to get all values from an associative array by mapping the array of arrays to an array of values.
Example
const obj = { a: 1, b: 2, c: 3 };
const values = Object.entries(obj).map(([key, value]) => value);
console.log(values); // Output: [1, 2, 3]
Note that the methods above will only work for associative arrays implemented using objects in JavaScript. If you have an array with numeric indices, you can simply use the Array.prototype.values() method to get an iterator of its values.
Example
const arr = [1, 2, 3];
const values = arr.values();
console.log([...values]); // Output: [1, 2, 3]