Remove Duplicates from an Array with JavaScript
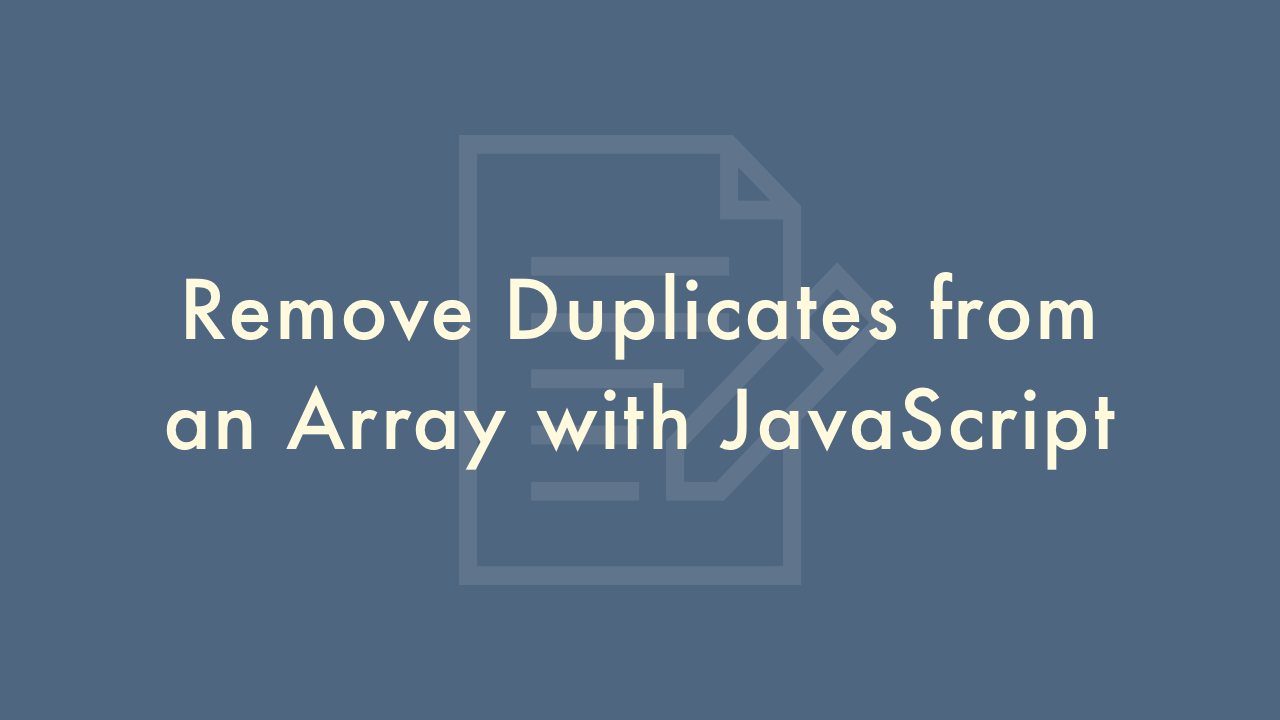
02/15/2022
Contents
In this article, you will learn how to remove duplicates from an array with JavaScript.
Remove duplicates from an array
There are several ways to remove duplicates from an array, but in JavaScript it’s relatively easy to implement using the filter() and indexOf() methods.
First, let’s review the filter() method and the indexOf method.
The filter() method
For an array, the filter() method calls the callback function in order, and returns only the element that returns true as the return value.
The filter() method can be written as below.
array.filter(callbackFn);
The indexOf() method
Use the indexOf() method to search an array for any element and get the index of the first element.
The indexOf() method can be written as below.
array.indexOf(searchElement);
Sample Code
Below is sample code that uses the filter() and indexOf() methods to remove duplicates from an array.
let array = [1,2,3,2,3,2,5,8,8,10];
array.filter(function (x, i, self) {
return self.indexOf(x) === i;
});
console.log(array); // [ 1, 2, 3, 5, 8, 10]