How to Pad a Number with Leading Zeros in JavaScript
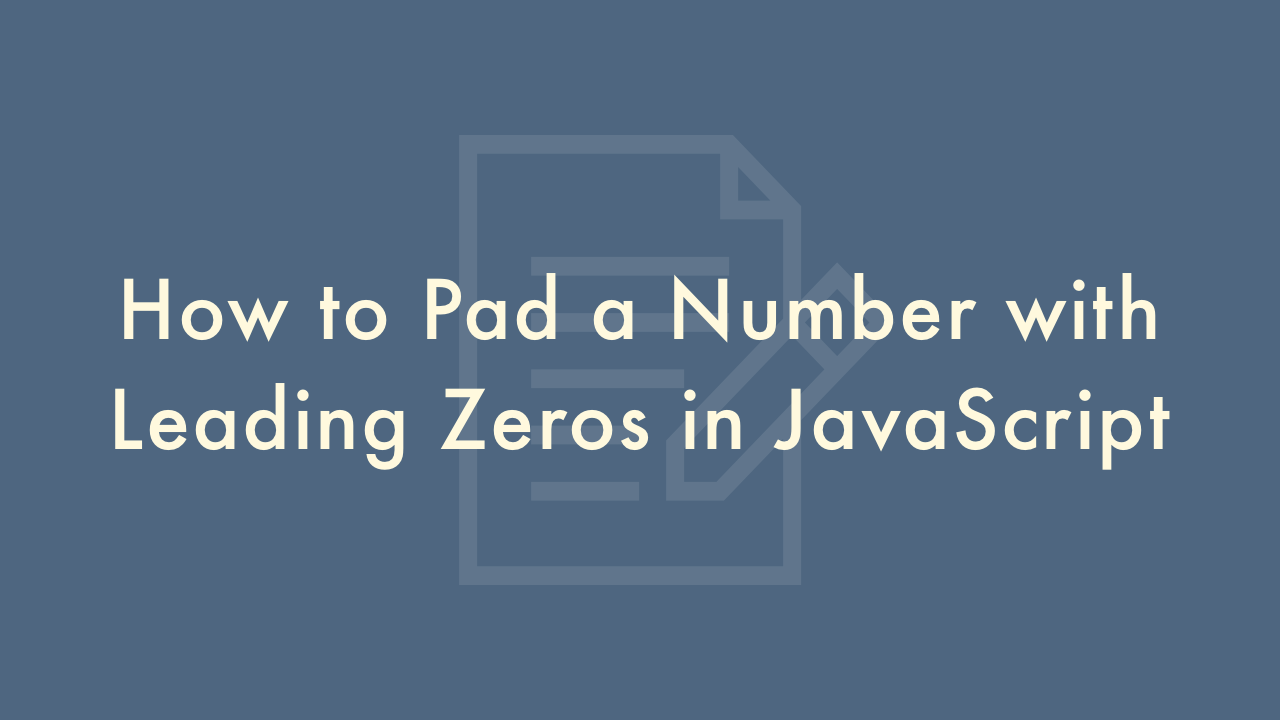
Contents
In this article, you will learn how to pad a number with leading zeros in JavaScript.
Padding a number with leading zeros in JavaScript
In JavaScript, you can pad a number with leading zeros to make it a certain number of digits long. Padding a number with leading zeros is useful when you need to display numbers with a consistent number of digits, such as when displaying dates or times.
Using the padStart() method
The padStart() method is a built-in method in JavaScript that pads a string with a specific character or characters until it reaches a specified length. Here’s the syntax for using the padStart() method to pad a number with leading zeros:
number.toString().padStart(length, "0")
The number is the number that you want to pad with leading zeros. The length is the total number of digits that the padded number should have. The “0” is the character that you want to use for padding the number.
Here’s an example of using the padStart() method to pad a number with leading zeros:
let number = 42;
let padded = number.toString().padStart(5, "0");
console.log(padded); // "00042"
In this example, we use the padStart() method to pad the number 42 with leading zeros so that it has a total of 5 digits. The resulting padded number is then assigned to the padded variable and logged to the console.
Using string concatenation
You can also pad a number with leading zeros using string concatenation. Here’s the syntax for using string concatenation to pad a number with leading zeros:
"0".repeat(length - number.toString().length) + number.toString()
The number is the number that you want to pad with leading zeros. The length is the total number of digits that the padded number should have.
Here’s an example of using string concatenation to pad a number with leading zeros:
let number = 42;
let padded = "0".repeat(5 - number.toString().length) + number.toString();
console.log(padded); // "00042"
In this example, we use string concatenation to pad the number 42 with leading zeros so that it has a total of 5 digits. The resulting padded number is then assigned to the padded variable and logged to the console.
Using the slice() method
You can also pad a number with leading zeros using the slice() method. Here’s the syntax for using the slice() method to pad a number with leading zeros:
("00000" + number).slice(-length)
The number is the number that you want to pad with leading zeros. The length is the total number of digits that the padded number should have.
Here’s an example of using the slice() method to pad a number with leading zeros:
let number = 42;
let padded = ("00000" + number).slice(-5);
console.log(padded); // "00042"
In this example, we use the slice() method to pad the number 42 with leading zeros so that it has a total of 5 digits. The resulting padded number is then assigned to the padded variable and logged to the console.