How to Use the Ruby dir.open Method
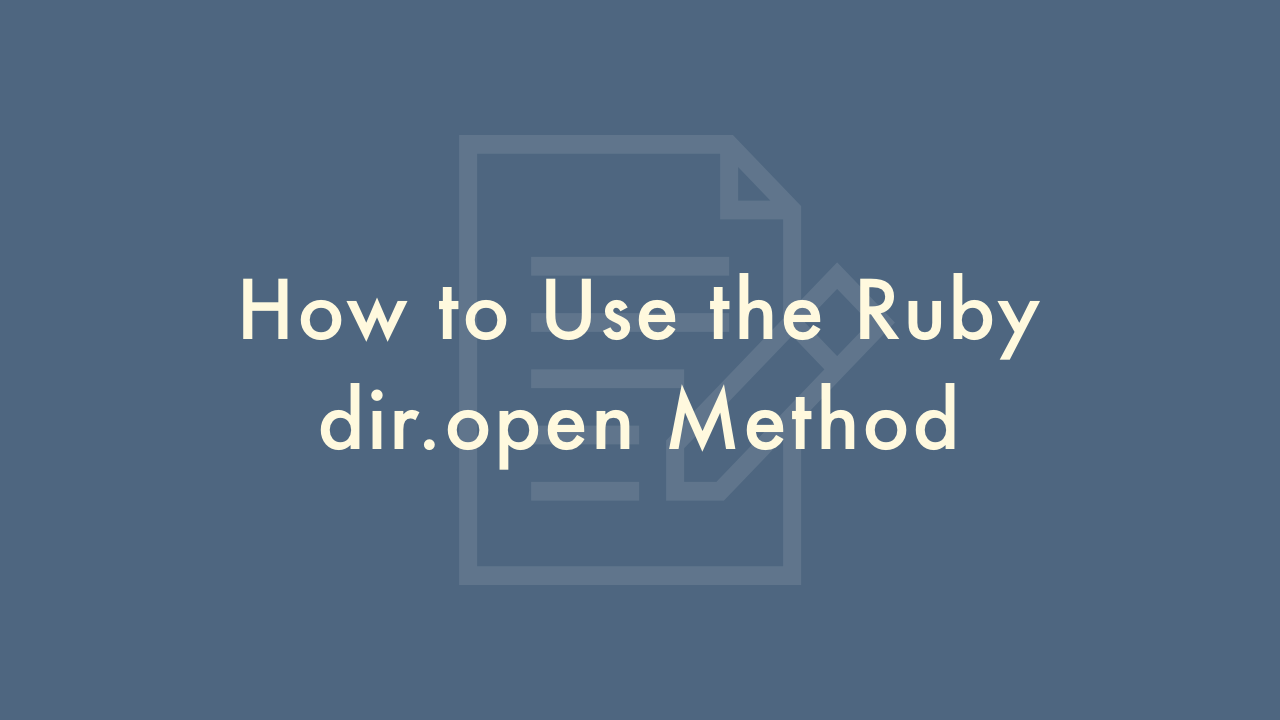
Contents
In this article, you will learn how to use the Ruby dir.open method.
Using the dir.open method
The dir.open method is a built-in method in Ruby that allows you to open a directory and read the contents of that directory. It returns a new Dir object that you can use to iterate over the files and directories in the specified directory. Here’s how to use the dir.open method:
dir = Dir.open('/path/to/directory')
This will create a new Dir object and open the directory located at the specified path. You can replace /path/to/directory with the actual path to the directory you want to open.
Once you have opened the directory, you can iterate over the files and directories it contains using the each method:
dir.each do |filename|
# Do something with filename
end
This will execute the code in the block for each file or directory in the directory. The filename variable will contain the name of the current file or directory being processed.
You can also use other methods provided by the Dir class to work with the files and directories in the directory. For example, you can use the rewind method to reset the directory to its original state:
dir.rewind
You can use the path method to get the path of the directory:
dir.path
You can use the close method to close the directory when you’re done working with it:
dir.close
Finally, it’s important to note that the dir.open method can also take a block. If you pass a block to the dir.open method, the block will be automatically closed when the block is exited:
Dir.open('/path/to/directory') do |dir|
dir.each do |filename|
# Do something with filename
end
end
In this example, the directory will be automatically closed when the block is exited, so you don’t need to call the close method manually.