Add Element to head Section with JavaScript
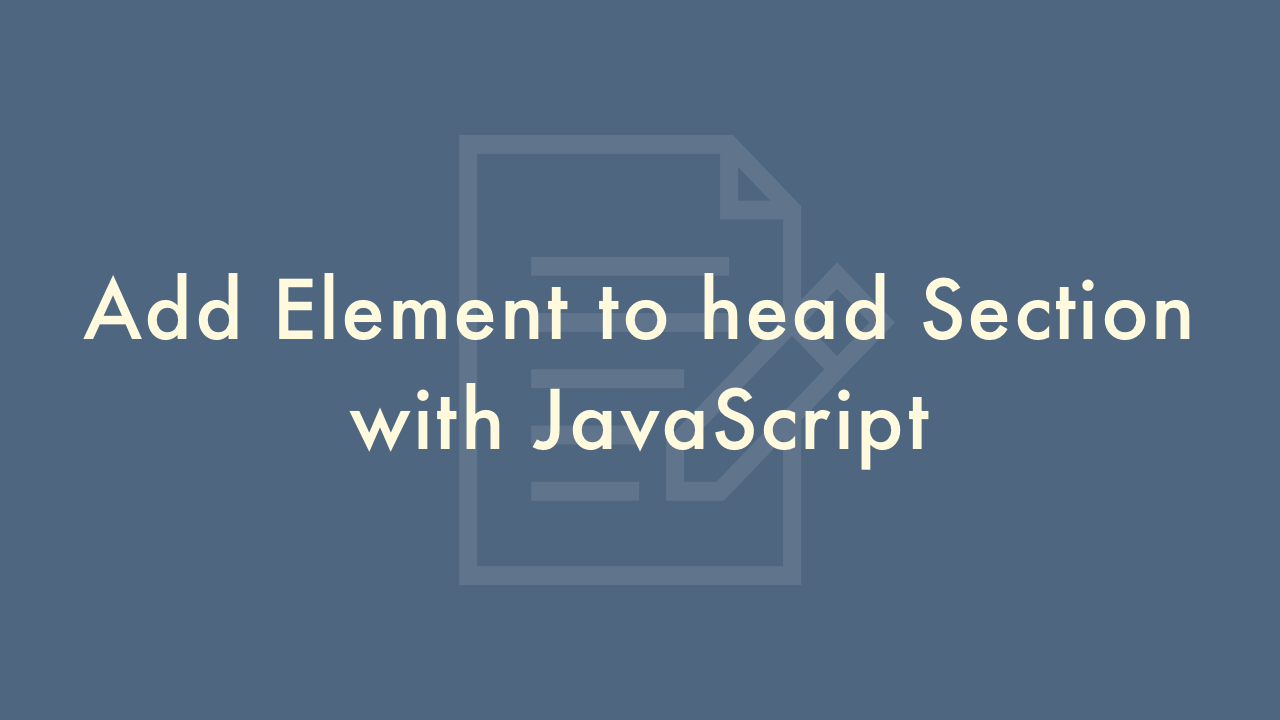
Contents
In this article, you will learn how to add element to head section with JavaScript.
Get the head element
There are several ways to get the head element in JavaScript, but the typical one is the head property of the document. The document.head is a property that returns the head element of the currently open document.
The syntax is as follows.
const head = document.head;
In the above I used document.head, but you can also get the head element with querySelector.
const head = document.querySelector('head');
Add a new element to the head element
This article uses insertAdjacentHTML, which allows you to specify the insertion position to some extent.
This time I want to add a new element inside the head element, so add it at the positions of afterBegin and beforeEnd. afterBegin adds a new element before the first child element and beforeEnd adds a new element after the last child element.
//Get the head element
const head = document.head;
//Add <title> before the first child element
head.insertAdjacentHTML('afterBegin', '<title>afterBegin</title>');
//Add <title> after the last child element
head.insertAdjacentHTML('beforeEnd', '<title>beforeEnd</title>');
//<head>
// <title>afterBegin</title>
// <meta charset="UTF-8">
// <meta name="description" content="">
// <title>beforeEnd</title>
//</head>
With the above method, you can get the head element with JavaScript and add a new element.