How to Use the Python bytes() Function
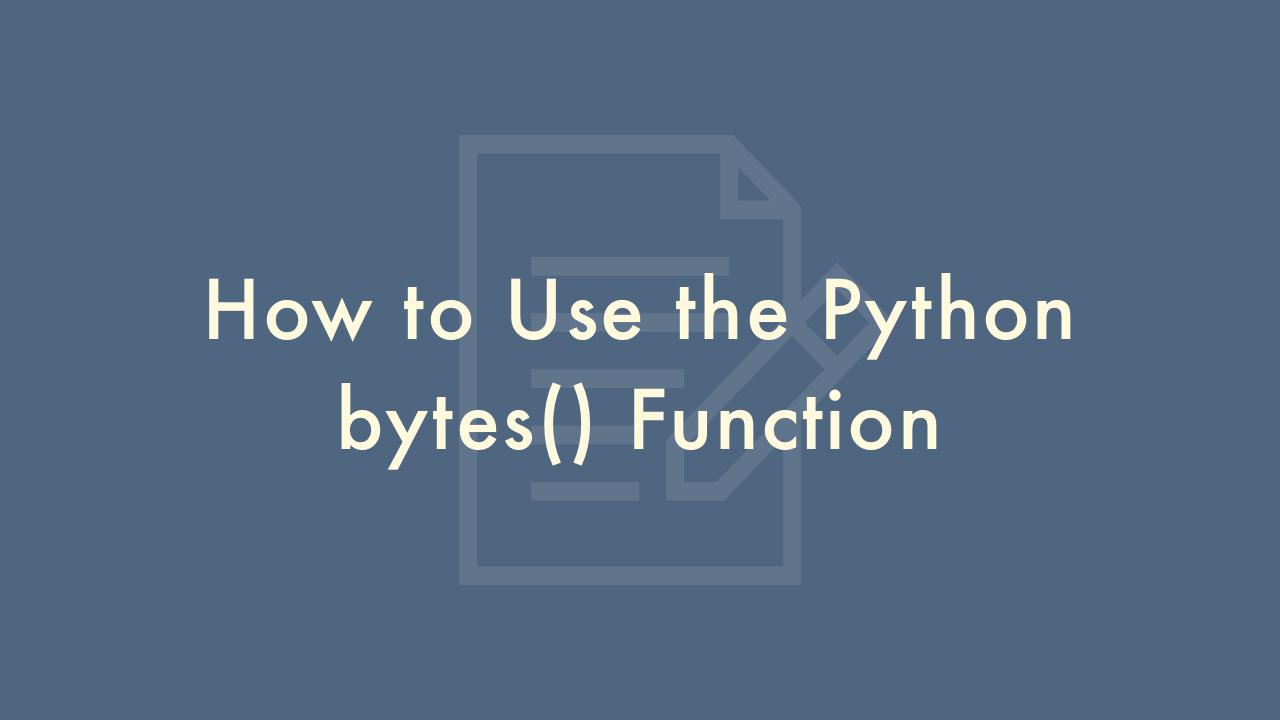
Contents
In this article, you will learn how to use the Python bytes() function.
Python bytes() Function
The bytes() function in Python is used to create a new immutable bytes object that contains the specified sequence of bytes. Here’s how you can use the bytes() function:
Creating a bytes object from a string:
You can create a bytes object from a string by encoding the string into bytes using a specified encoding scheme. For example, to create a bytes object from the string “Hello, world!” using the UTF-8 encoding scheme, you can do the following:
my_bytes = bytes("Hello, world!", "utf-8")
Creating a bytes object from a list of integers:
You can also create a bytes object from a list of integers representing the byte values. For example, to create a bytes object from the list [72, 101, 108, 108, 111, 44, 32, 119, 111, 114, 108, 100, 33], you can do the following:
my_bytes = bytes([72, 101, 108, 108, 111, 44, 32, 119, 111, 114, 108, 100, 33])
Creating a bytes object from a bytes-like object:
You can also create a bytes object from a bytes-like object, such as a bytearray or memoryview. For example, to create a bytes object from a bytearray object my_bytearray, you can do the following:
my_bytes = bytes(my_bytearray)
Note that the bytes() function takes a second optional parameter that specifies the encoding scheme to use when creating a bytes object from a string. If this parameter is not specified, the default encoding scheme is used.
Also, it’s important to note that bytes objects are immutable, which means you cannot modify the bytes in a bytes object once it’s created. If you need a mutable sequence of bytes, you should use a bytearray object instead.