How to Use the Ruby Date.today Method
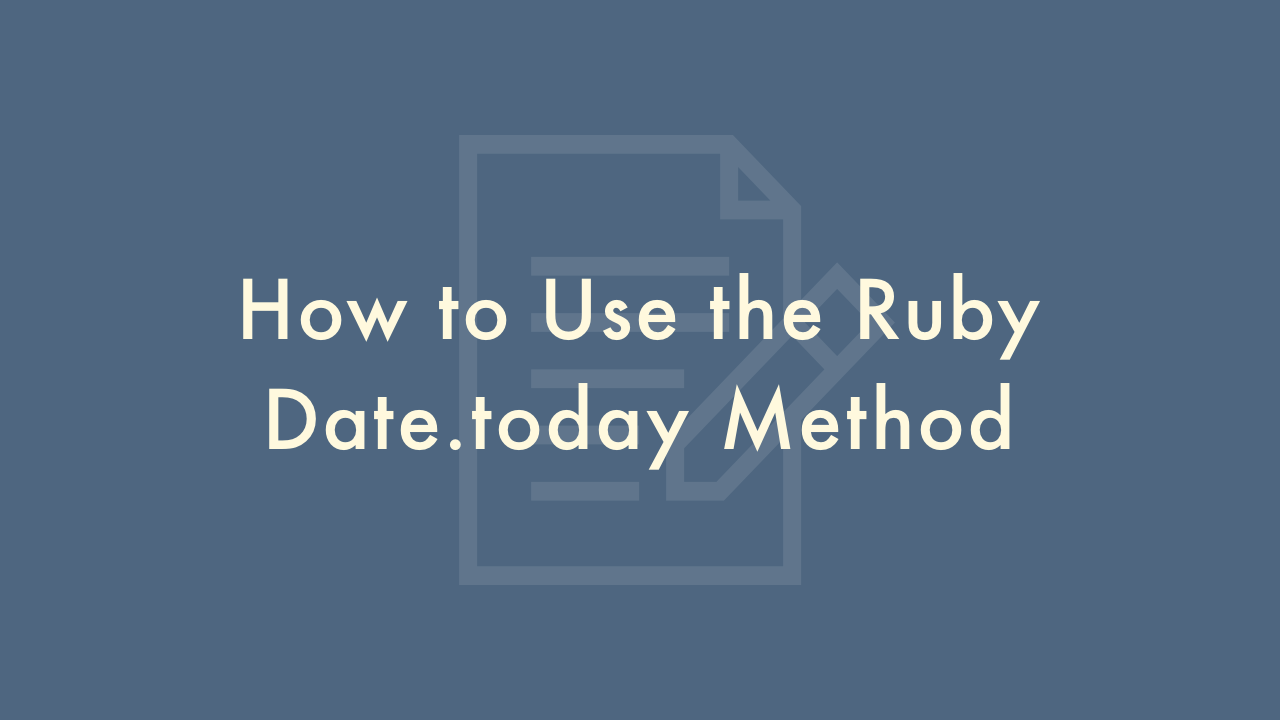
Contents
In this article, you will learn how to use the Ruby Date.today method.
Using the Date.today method
The Ruby programming language provides a built-in Date class that allows you to work with dates and times. One of the most commonly used methods of the Date class is Date.today, which returns the current date in your system’s timezone.
Here’s how you can use the Date.today method in your Ruby programs:
require 'date'
today = Date.today
puts today
In the above example, we first require the Date class using the require keyword. This is necessary because the Date class is not part of the core Ruby library, so we need to explicitly load it.
Next, we call the Date.today method, which returns an instance of the Date class representing the current date. We assign this instance to a variable called today.
Finally, we use the puts method to print the today variable to the console. When you run this program, you should see today’s date printed in the format YYYY-MM-DD.
You can also use the Date.today method to perform date calculations. For example, let’s say you want to find out what day of the week it will be 100 days from now. Here’s how you can do it:
require 'date'
today = Date.today
future_date = today + 100
puts future_date.strftime("%A")
In this example, we first create a variable called today and assign it the current date using the Date.today method.
Next, we create another variable called future_date and assign it the value of today + 100. This performs a date calculation and adds 100 days to the today variable. The result is a new instance of the Date class representing the date 100 days in the future.
Finally, we use the strftime method to format the future_date as a string using the %A format specifier. This specifier returns the full name of the day of the week (e.g. “Monday”, “Tuesday”, etc.). We then print the result to the console using the puts method.
When you run this program, you should see the name of the day of the week that is 100 days from now printed to the console.