Get and Set Data Attributes with JavaScript dataset
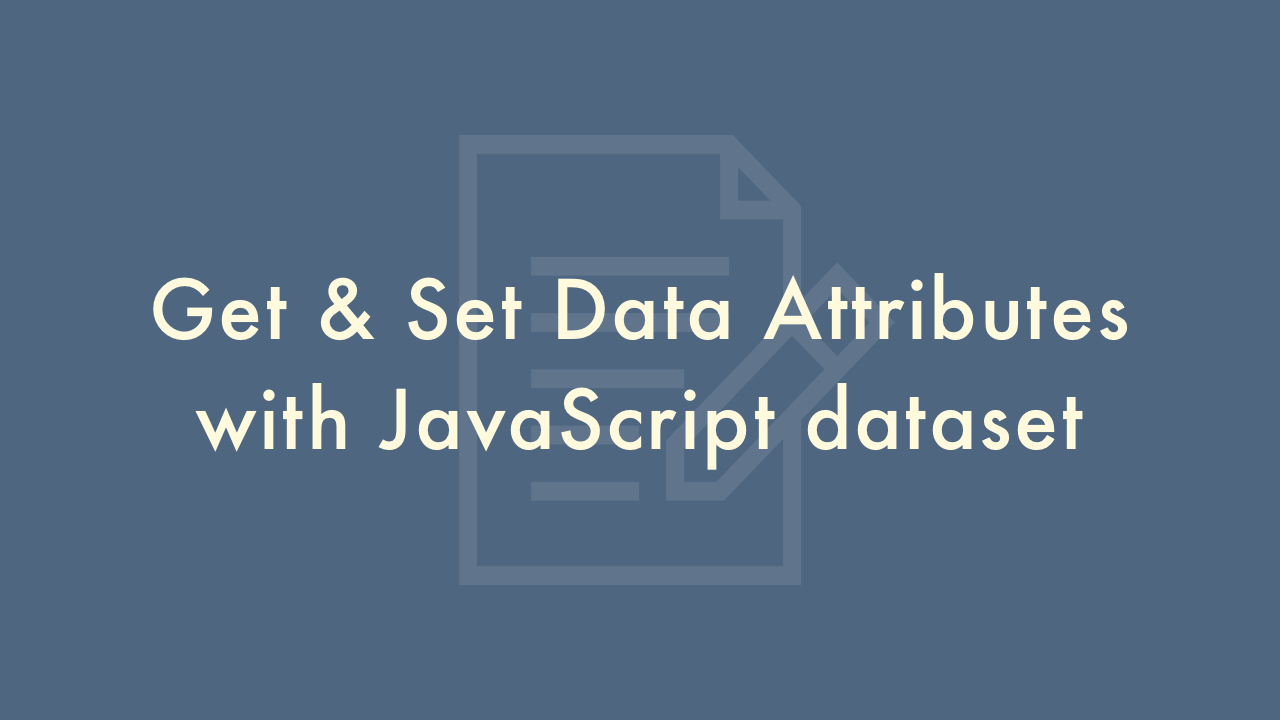
Contents
In this article, you will learn how to get and set custom data attributes (data-*) with JavaScript dataset property.
What is dataset?
To get and set custom data attributes (data-*) on HTML elements, you can use the JavaScript dataset property.
This property is read-only and exposes a map of strings (DOMStringMap) with an entry for each data-* attribute.
It is possible to access custom data attributes (data-*) using the getAttribute and setAttribute properties, but the dataset property is simpler to write and seems to have better performance.
Get custom data attributes (data-*)
Try to get the custom data attributes from the HTML below.
<div id="myid"
data-user-name="Plantpot"
data-country="Japan"
data-city="Tokyo"
data-lang="ja"
>
</div>
First, the following code is an example to get all the custom data attributes of the specified element.
const element = document.getElementById('myid');
console.log(element.dataset);
/*
{
city: "Tokyo"
country: "Japan"
lang: "ja"
userName: "Plantpot"
}
*/
Second, the following code is an example to get an individual data attribute of the specified element.
const element = document.getElementById('myid');
console.log(element.dataset.userName); // Plantpot
console.log(element.dataset.country); // Japan
console.log(element.dataset.city); // Tokyo
console.log(element.dataset.lang); // ja
Set custom data attributes (data-*)
The following code is an example to create a new custom data attribute on the specified element.
const element = document.getElementById('myid');
element.dataset.myData = "foo bar";
console.log(element.dataset.myData); // foo bar
The HTML will be updated like this.
<div id="myid"
data-user-name="Plantpot"
data-country="Japan"
data-city="Tokyo"
data-lang="ja"
data-my-data="foo bar"
>
</div>
Update custom data attributes (data-*)
The following code is an example to update the custom data attributes on the specified element.
const element = document.getElementById('myid');
element.dataset.userName = "test";
console.log(element.dataset.userName); // test
element.dataset.country = "United States";
console.log(element.dataset.country); // United States
element.dataset.city = "New York";
console.log(element.dataset.city); // New York
element.dataset.lang = "en-us";
console.log(element.dataset.lang); // en-us
The HTML will be updated like this.
<div id="myid"
data-user-name="test"
data-country="United States"
data-city="New York"
data-lang="en-us"
>
</div>
Delete custom data attributes (data-*)
The following code is an example to delete the custom data attributes from the specified element.
const element = document.getElementById('myid');
delete element.dataset.userName;
console.log(element.dataset.userName) // undefined
The HTML will be updated like this.
<div id="myid"
data-country="Japan"
data-city="Tokyo"
data-lang="ja"
>
</div>