How to Get CSS Styles of an DOM Element in JavaScript
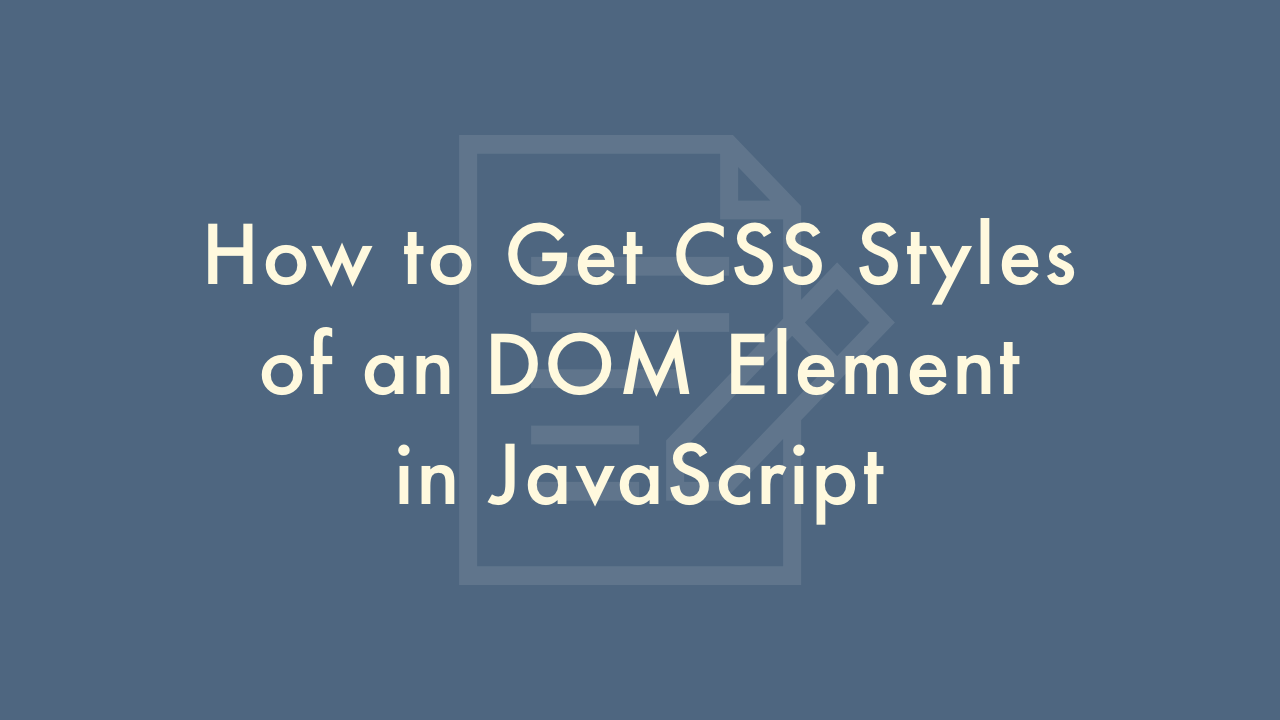
Contents
In this article, you will learn how to get CSS styles of an DOM element in JavaScript.
Getting CSS styles of an DOM element in JavaScript
In JavaScript, you can get the CSS styles of a DOM element using the getComputedStyle() method. This method returns an object that contains the computed style values for the specified element.
Example
const element = document.querySelector('.my-class');
const styles = window.getComputedStyle(element);
console.log(styles.color); // Output: rgb(0, 0, 0)
console.log(styles.fontSize); // Output: 16px
This example first gets the element using the querySelector() method, and then gets its computed styles using the getComputedStyle() method. The styles variable is then set to an object that contains the computed style values for the element. You can access specific style values by using the property names of the styles object.
It’s important to note that the getComputedStyle() method returns computed styles, which may be different from the inline styles that are defined in the HTML markup or in a CSS file. Computed styles are the result of applying all CSS rules that affect the element, including inherited styles and styles defined in media queries.
You can also get the value of a specific CSS property for an element using the getPropertyValue() method of the CSSStyleDeclaration interface.
Example
const element = document.querySelector('.my-class');
const styles = window.getComputedStyle(element);
const color = styles.getPropertyValue('color');
console.log(color); // Output: rgb(0, 0, 0)
This example first gets the element and its computed styles using the getComputedStyle() method. The getPropertyValue() method is then called with the name of the CSS property as an argument to get the value of the color property.