How to Use attr_accessor in Ruby
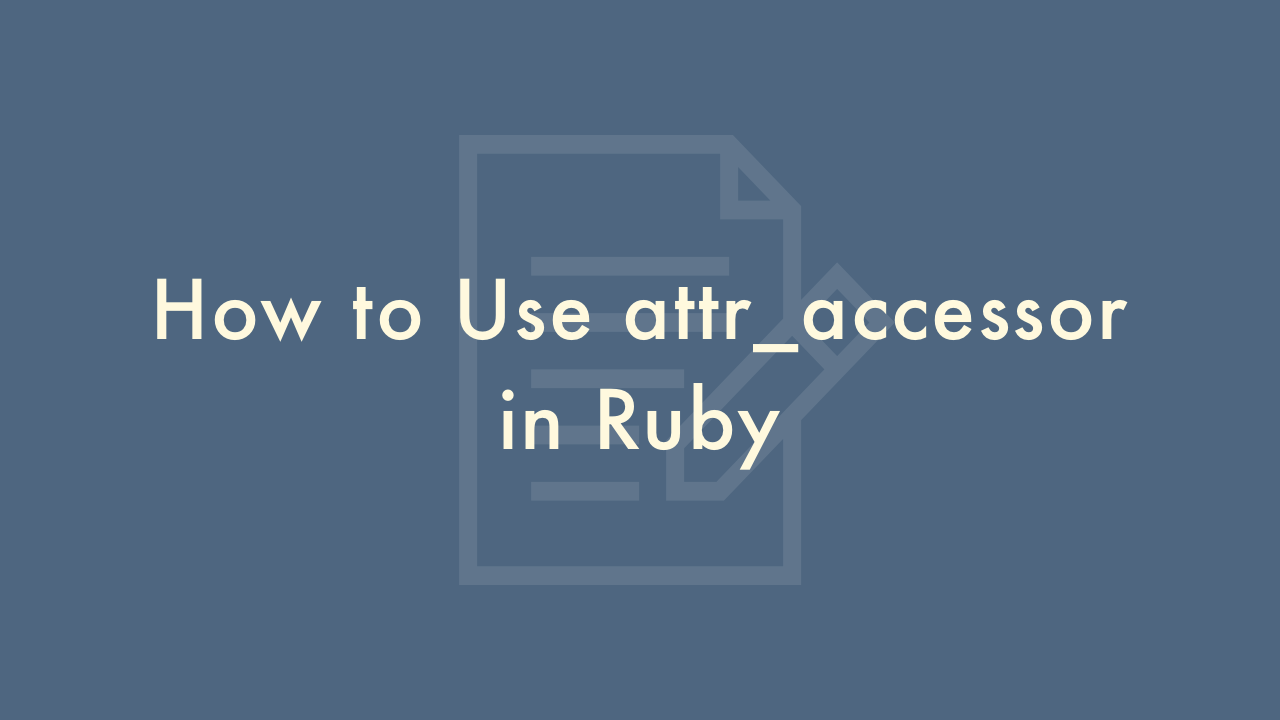
Contents
In this article, you will learn how to use attr_accessor in Ruby.
Using attr_accessor
In Ruby, attr_accessor is a shorthand for creating getter and setter methods for a class instance variable. It is used to define an attribute with both read and write capabilities.
Here is an example:
class Person
attr_accessor :name
def initialize(name)
@name = name
end
end
In the example above, attr_accessor is used to define the name attribute. This creates both a getter and setter method for the instance variable @name.
We can now create an instance of the Person class and use the name attribute:
person = Person.new("John")
puts person.name #=> "John"
person.name = "Jane"
puts person.name #=> "Jane"
In the above example, we create a new Person object with the name “John” and use the name getter method to output the name. We then set the name to “Jane” using the name= setter method and output the name again to confirm that it has been updated.
Here are some more details about attr_accessor in Ruby:
- attr_accessor is actually a combination of two other methods, attr_reader and attr_writer. attr_reader creates only the getter method for an instance variable, while attr_writer creates only the setter method. attr_accessor combines these two methods to create both getter and setter methods.
-
You can use attr_accessor to define multiple attributes at once, separated by commas:
class Person attr_accessor :name, :age, :gender end
- attr_accessor works by creating instance variables for each attribute you define. For example, in the above Person class, attr_accessor :name creates an instance variable @name that can be accessed by the getter and setter methods.
-
You can also define attributes using attr_reader and attr_writer separately, if you only need one of the methods:
class Person attr_reader :name attr_writer :age end
- attr_accessor is commonly used in object-oriented programming to create objects with attributes that can be accessed and modified externally. This makes it easier to create objects that can interact with other objects and systems.