How to Get Stock Price Data with Python pandas
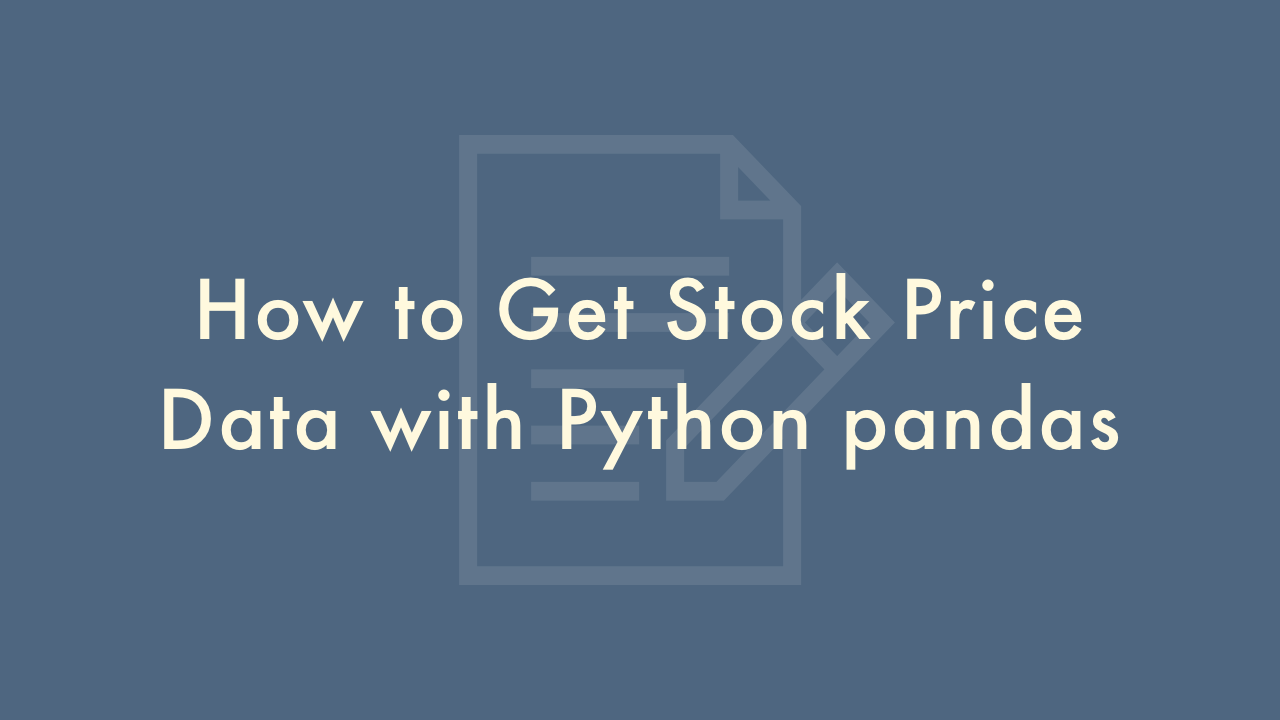
09/17/2021
Contents
In this article, you will learn how to get stock price data with Python pandas.
Get Stock Price Data with Python pandas
To get stock price data with Python pandas, you can use the pandas-datareader library. This library allows you to download historical stock price data from a variety of sources, including Yahoo Finance, Google Finance, and more.
Here’s a step-by-step guide on how to use pandas-datareader to get stock price data:
Install pandas-datareader library:
!pip install pandas-datareader
Import the necessary libraries:
import pandas_datareader as pdr
import datetime
Define the stock symbol and the date range for which you want to retrieve data:
stock_symbol = 'AAPL'
start_date = datetime.datetime(2020, 1, 1)
end_date = datetime.datetime(2021, 1, 1)
Use the DataReader function from pandas-datareader to download the data:
stock_data = pdr.DataReader(stock_symbol, 'yahoo', start_date, end_date)
In this example, we are using Yahoo Finance as our data source. If you want to use a different source, you can replace ‘yahoo’ with the appropriate data source code.
The stock_data variable now contains a pandas DataFrame with the stock price data for the specified date range:
print(stock_data.head())
This will print the first five rows of the DataFrame:
High Low Open Close Volume Adj Close
Date
2020-01-02 75.150002 73.797501 74.059998 75.087502 135480400.0 73.840042
2020-01-03 75.144997 74.125000 74.287498 74.357498 146322800.0 73.122154
2020-01-06 74.989998 73.187500 73.447502 74.949997 118387200.0 73.704819
2020-01-07 75.224998 74.370003 74.959999 74.597504 108872000.0 73.358185
2020-01-08 76.110001 74.290001 74.290001 75.797501 132079200.0 74.537514