How to Compare Two Strings with the PHP strcmp() Function
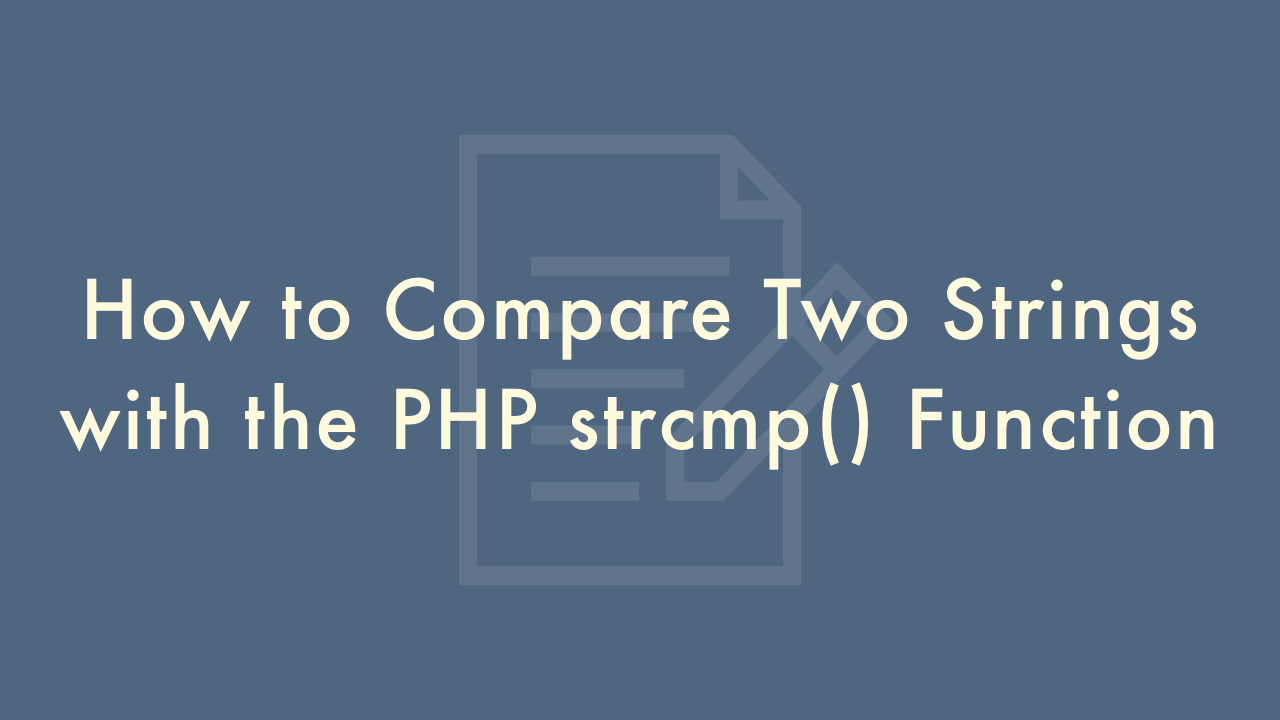
Contents
In this article, you will learn how to compare two strings with the PHP strcmp() function.
PHP strcmp() Function
The strcmp() function in PHP is used to compare two strings. It returns an integer value indicating the result of the comparison. A value of 0 indicates that the strings are equal, a value less than 0 indicates that the first string is less than the second, and a value greater than 0 indicates that the first string is greater than the second.
In more detail, the strcmp() function is a built-in function in PHP that compares two strings lexicographically. It compares each character of the strings one by one, starting with the first character of each string. If the first character of the first string is smaller than the first character of the second string, the function returns a negative number. If the first character of the first string is greater than the first character of the second string, the function returns a positive number. If the first characters of both strings are equal, the function compares the second characters, and so on, until it finds a difference or reaches the end of one of the strings. If the function reaches the end of both strings and all the characters are equal, it returns 0, indicating that the strings are equal.
The basic syntax for using the strcmp() function is:
strcmp ($string1 , $string2)
Here, $string1
and $string2
are the two strings that you want to compare.
For example:
<?php
$string1 = "Hello";
$string2 = "world";
$result = strcmp($string1, $string2);
if ($result < 0) {
echo "\"$string1\" is less than \"$string2\"";
} elseif ($result > 0) {
echo "\"$string1\" is greater than \"$string2\"";
} else {
echo "\"$string1\" is equal to \"$string2\"";
}
?>
output:
"Hello" is less than "world"
It’s important to note that strcmp() compares the strings based on the ASCII value of each character, so it is case-sensitive. If you want to compare strings in a case-insensitive manner, you can use the strcasecmp() function instead, which compares strings based on the ASCII value of each character, but ignores the case of the characters.