How to Create a User-Defined Function in MySQL
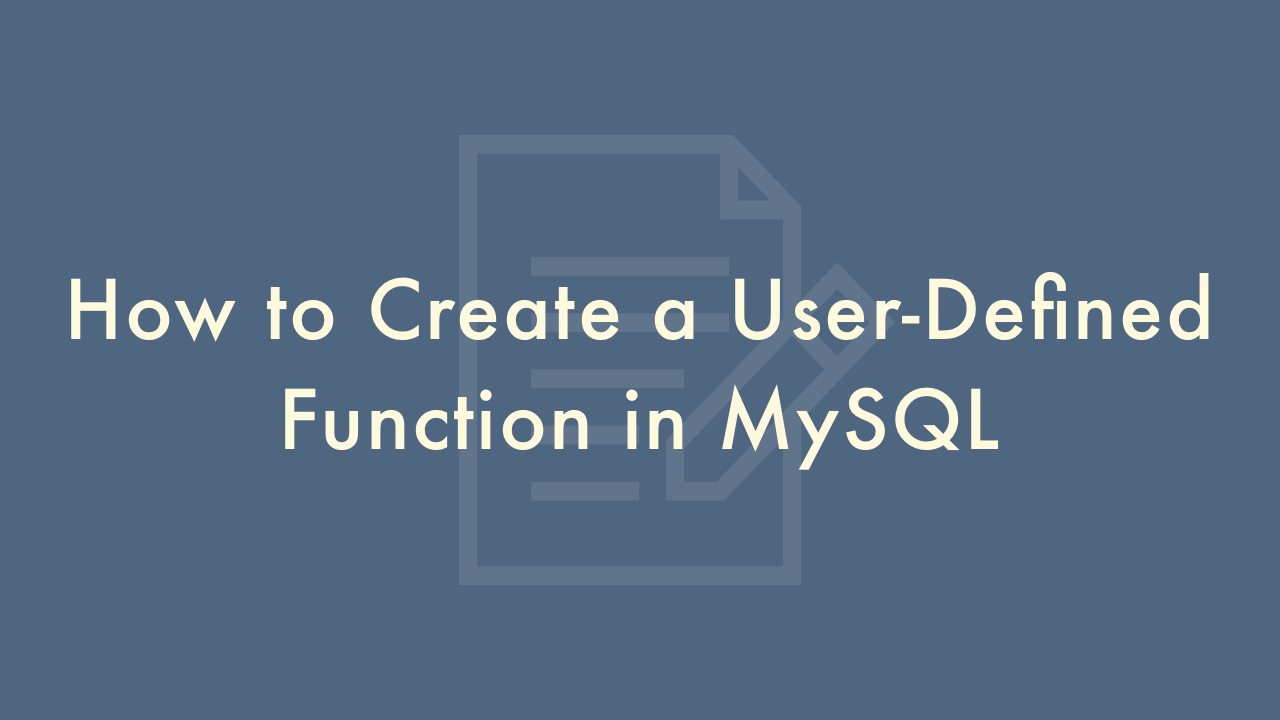
Contents
In this article, you will learn how to create a user-defined function in MySQL.
Creating a user-defined function in MySQL
MySQL is a popular open-source relational database management system used to store and manage data. One of its key features is the ability to create user-defined functions, which are custom functions that can be used to perform specific tasks.
Create a database
The first step in creating a user-defined function in MySQL is to create a database to store the function. You can create a database using the following SQL statement:
CREATE DATABASE my_database;
Create a function
Once you have created a database, you can create a function using the following syntax:
CREATE FUNCTION function_name (parameter1 datatype, parameter2 datatype, ...) RETURNS return_datatype
BEGIN
-- Function body goes here
END;
Let’s take an example. Suppose we want to create a function that adds two numbers and returns the result. We can create the function as follows:
CREATE FUNCTION add_numbers (num1 INT, num2 INT) RETURNS INT
BEGIN
DECLARE result INT;
SET result = num1 + num2;
RETURN result;
END;
In the above example, we created a function called “add_numbers” that takes two parameters, “num1” and “num2”, and returns an integer value. The function adds the two numbers and assigns the result to a variable called “result”. Finally, the function returns the value of the “result” variable.
Use the function
Once you have created a user-defined function in MySQL, you can use it in your SQL queries just like any other built-in function. For example, to use the “add_numbers” function we created earlier, we can write the following SQL statement:
SELECT add_numbers(10, 20);
The above SQL statement will return the value 30, which is the result of adding 10 and 20 using the “add_numbers” function.