How to Get the Index of the Selected Option in a Select Box in JavaScript
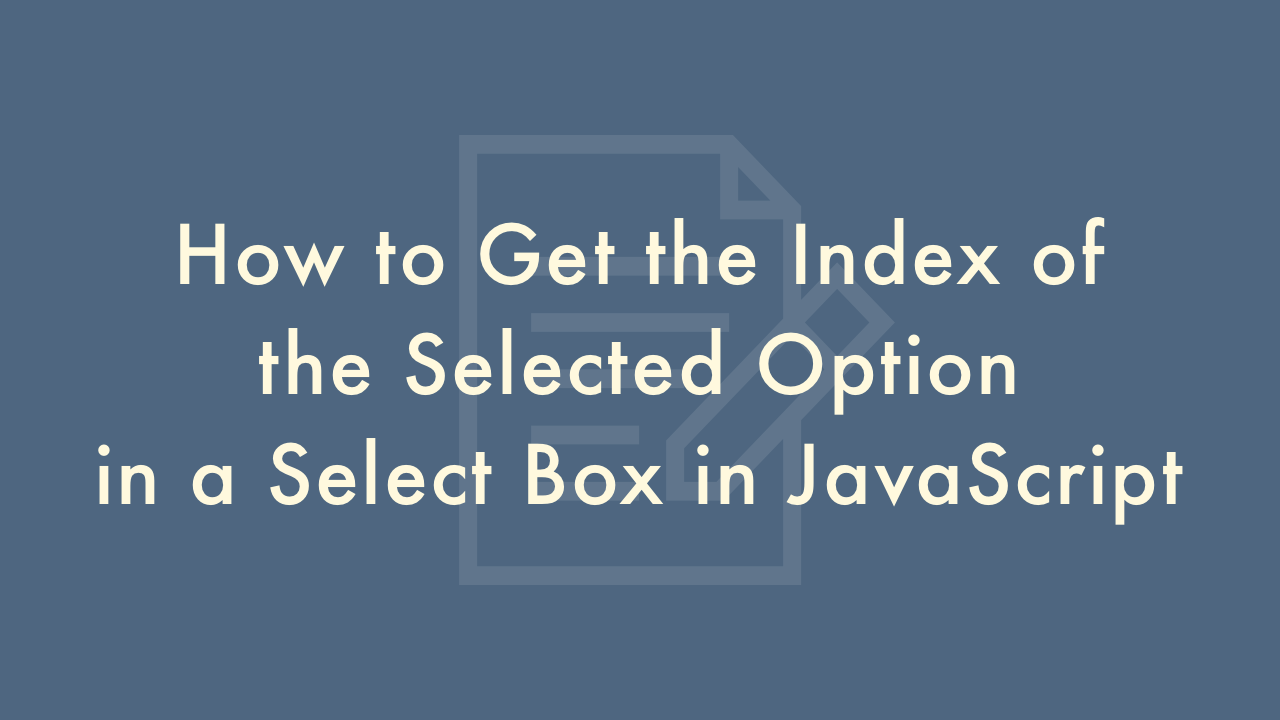
Contents
In this article, you will learn how to get the index of the selected option in a select box in JavaScript.
Getting the index of the selected option in a select box in JavaScript
In JavaScript, you can get the index of the selected option in a select box using the selectedIndex property of the HTMLSelectElement interface.
Example
const selectBox = document.getElementById('my-select-box');
const selectedIndex = selectBox.selectedIndex;
console.log(selectedIndex); // Output: 2
This example first gets the select box element using the getElementById() method, and then gets its selected index using the selectedIndex property. The selectedIndex property returns the index of the selected option, starting from zero.
If you want to get the value of the selected option instead of its index, you can use the value property of the HTMLSelectElement interface.
Example
const selectBox = document.getElementById('my-select-box');
const selectedValue = selectBox.value;
console.log(selectedValue); // Output: "option-3"
This example first gets the select box element using the getElementById() method, and then gets its selected value using the value property. The value property returns the value of the selected option.
It’s important to note that if the select box has the multiple attribute set, the selectedIndex and value properties will return the index and value of the first selected option. To get the index and value of all selected options, you can use the selectedOptions property of the HTMLSelectElement interface.
Example
const selectBox = document.getElementById('my-select-box');
const selectedOptions = selectBox.selectedOptions;
for (let i = 0; i < selectedOptions.length; i++) {
console.log(selectedOptions[i].index); // Output: 2, 4
console.log(selectedOptions[i].value); // Output: "option-3", "option-5"
}
This example first gets the select box element using the getElementById() method, and then gets its selected options using the selectedOptions property. The selectedOptions property returns a list of all selected options, each of which has an index and value property.