How to Find the Intersection Point of Two Lines in JavaScript
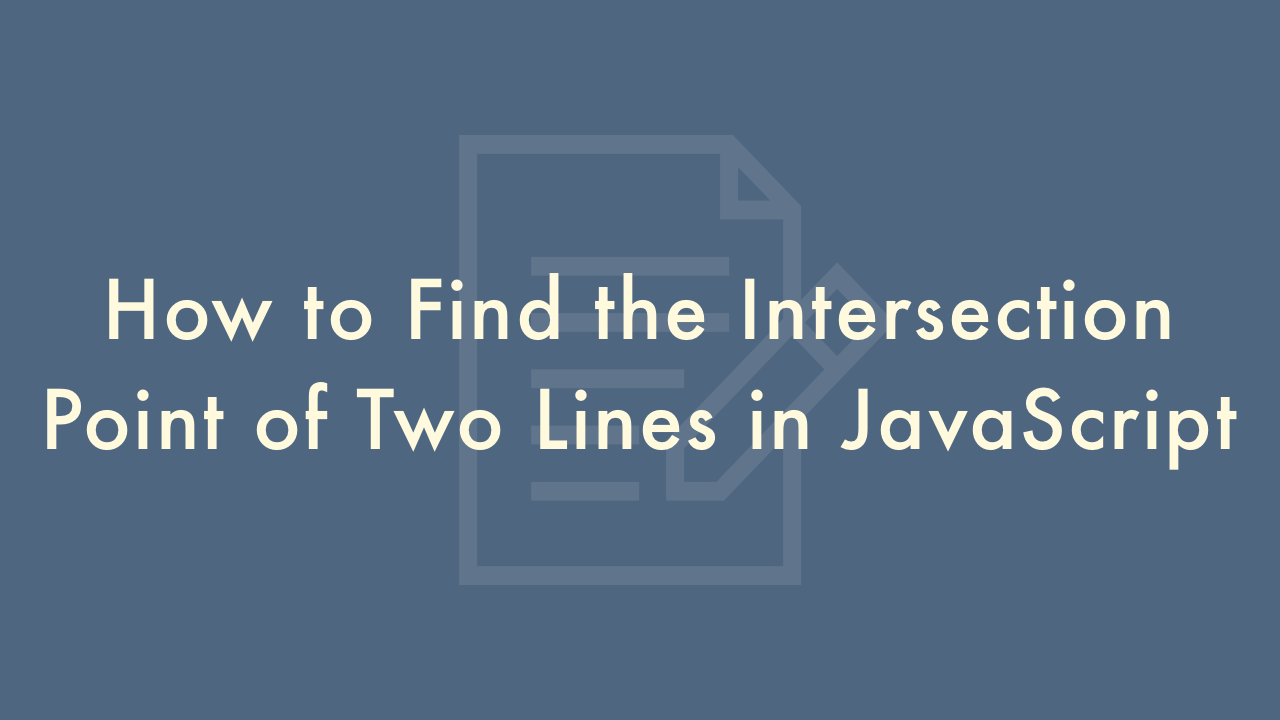
Contents
In this article, you will learn how to find the intersection point of two lines in JavaScript.
Finding the intersection point of two lines in JavaScript
To find the intersection point of two lines in JavaScript, we need to determine the equations of the lines and then solve for the point where they intersect. Here are the steps to accomplish this:
- Find the slope and y-intercept of each line.
- Set the two equations equal to each other and solve for x.
- Plug the value of x into either of the equations to find the y-value.
- Return the (x, y) coordinates of the intersection point.
Examples
Here is an example of how to use these steps in JavaScript:
function findIntersection(line1, line2) {
const slope1 = (line1.y2 - line1.y1) / (line1.x2 - line1.x1);
const yIntercept1 = line1.y1 - slope1 * line1.x1;
const slope2 = (line2.y2 - line2.y1) / (line2.x2 - line2.x1);
const yIntercept2 = line2.y1 - slope2 * line2.x1;
const x = (yIntercept2 - yIntercept1) / (slope1 - slope2);
const y = slope1 * x + yIntercept1;
return { x, y };
}
In this example, we define a findIntersection function that takes two line objects as arguments. Each line object should have the x1, y1, x2, and y2 properties, which represent the coordinates of the two points that define the line.
Inside the function, we first calculate the slope and y-intercept of each line using the formula y = mx + b, where m is the slope and b is the y-intercept. We use the two-point formula to find the slope and then solve for the y-intercept by plugging in one of the points and the slope.
Next, we set the two equations equal to each other and solve for x. This gives us the x-coordinate of the intersection point.
Finally, we plug the x-coordinate back into either of the equations to find the y-coordinate of the intersection point.
We return an object with the x and y coordinates of the intersection point.
Here is an example of how to use this function:
const line1 = { x1: 1, y1: 2, x2: 4, y2: 6 };
const line2 = { x1: 2, y1: 1, x2: 6, y2: 4 };
const intersection = findIntersection(line1, line2);
console.log(intersection); // Output: { x: 3, y: 4 }
In this example, we define two line objects, line1 and line2, with the coordinates of their endpoints. We then call the findIntersection function with these two lines as arguments and store the result in the intersection variable.
We log the intersection point to the console, which should be { x: 3, y: 4 }.
Note that this function assumes that the two lines intersect at exactly one point. If the lines are parallel or coincident, this function may return unexpected results.