The Cross-Table Update in MySQL
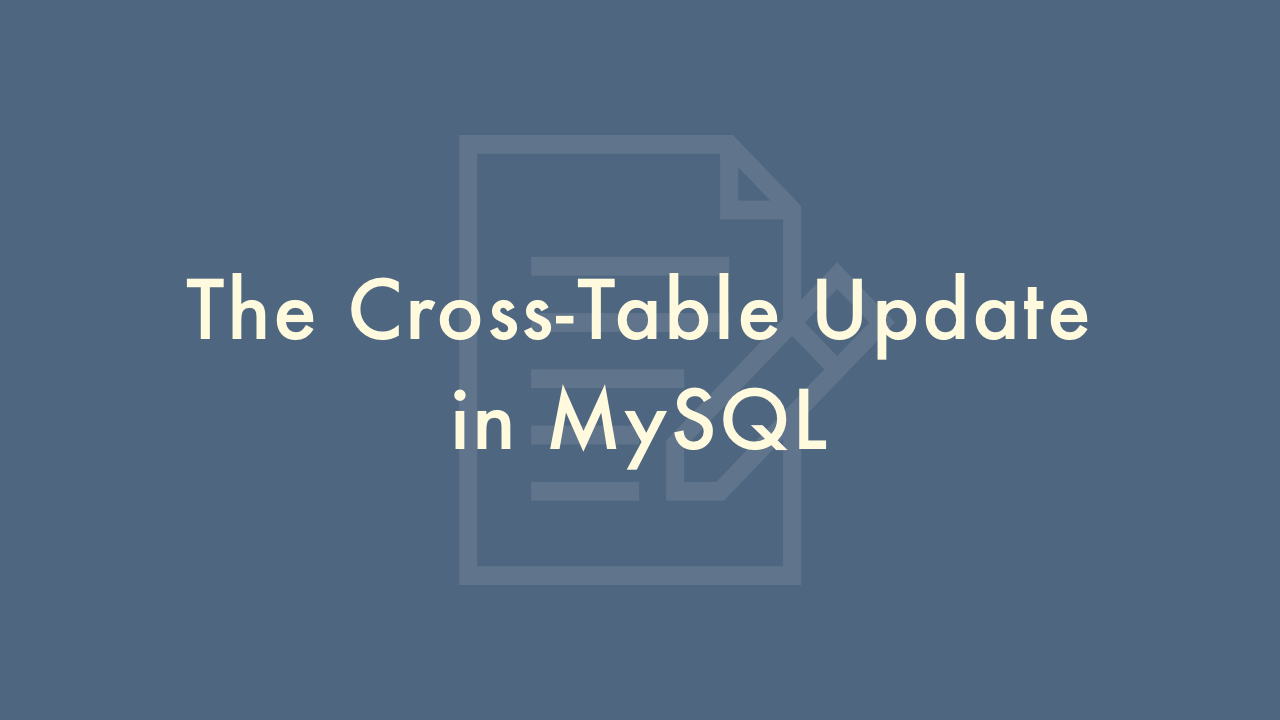
Contents
In this article, you will learn about the cross-table update in MySQL.
The cross-table update in MySQL
Cross-table update is a powerful feature in MySQL that allows you to update one table based on the values of another table. This can be useful in situations where you need to synchronize data between two tables or when you need to update data in one table based on the data in another table.
Here’s how to perform a cross-table update in MySQL in a few simple steps:
Create the two tables
You first need to create the two tables that you want to update. Let’s call them Table A and Table B. Table A is the table you want to update, and Table B is the table that contains the values you want to use for the update.
Identify the common field
You need to identify a common field between the two tables that you can use to join them. For example, if both tables have a “customer_id” field, you can use that field to join them.
Write the UPDATE statement
Now you can write the UPDATE statement that will update Table A based on the values in Table B. Here’s an example:
UPDATE table_a
JOIN table_b
ON table_a.customer_id = table_b.customer_id
SET table_a.balance = table_b.balance
This statement updates the “balance” field in Table A with the corresponding value from Table B, based on the “customer_id” field.
Execute the statement
Finally, you can execute the UPDATE statement to update Table A with the values from Table B.
Here’s an example of a complete cross-table update in MySQL:
CREATE TABLE table_a (
customer_id INT PRIMARY KEY,
name VARCHAR(255),
balance DECIMAL(10,2)
);
CREATE TABLE table_b (
customer_id INT PRIMARY KEY,
balance DECIMAL(10,2)
);
INSERT INTO table_a (customer_id, name, balance)
VALUES (1, 'John Smith', 100.00),
(2, 'Jane Doe', 50.00),
(3, 'Bob Johnson', 75.00);
INSERT INTO table_b (customer_id, balance)
VALUES (1, 150.00),
(2, 75.00),
(3, 100.00);
UPDATE table_a
JOIN table_b
ON table_a.customer_id = table_b.customer_id
SET table_a.balance = table_b.balance;
SELECT * FROM table_a;
This example creates two tables, Table A and Table B, inserts some sample data into them, and then performs a cross-table update to update the “balance” field in Table A with the corresponding value from Table B. Finally, it selects all the rows from Table A to show the updated data.