How to Use the PHP get_object_vars() Function
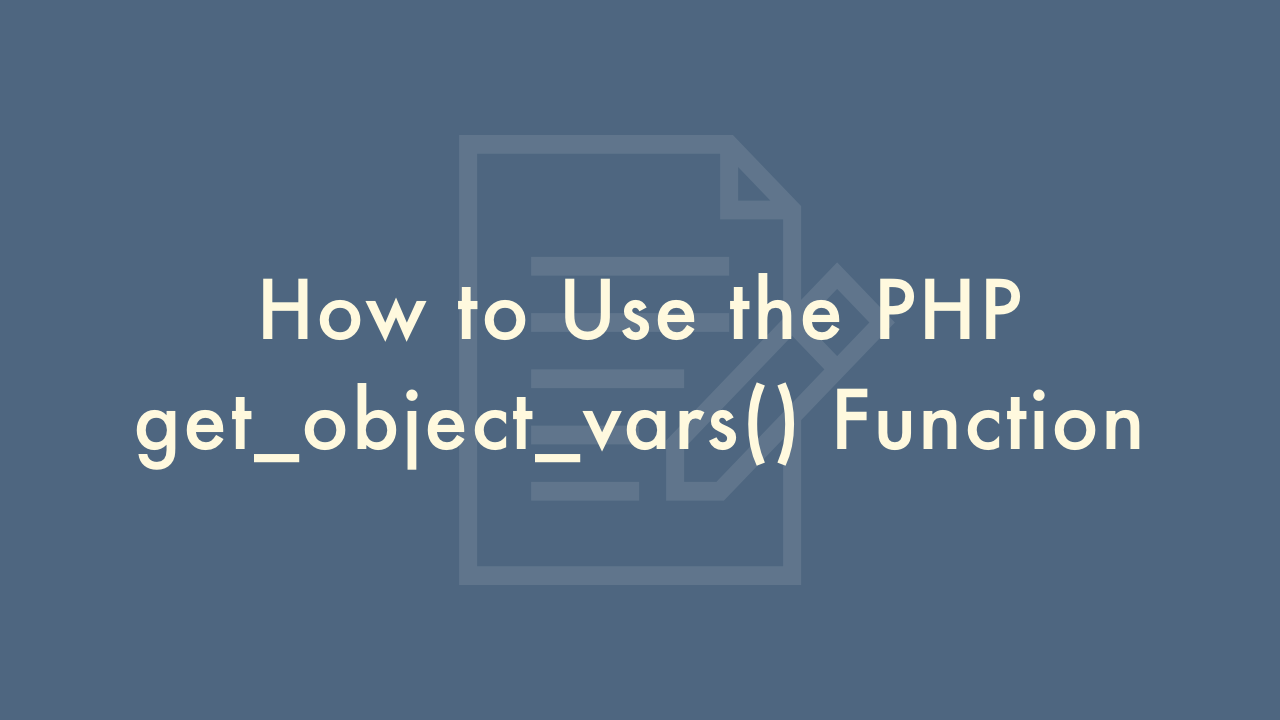
Contents
In this article, you will learn how to use the PHP get_object_vars() function.
PHP get_object_vars() Function
The get_object_vars() function in PHP is used to retrieve the properties of an object as an associative array.
Syntax:
array get_object_vars ( object $object )
Parameters:
$object
: The object for which you want to retrieve the public properties.
Example:
<?php
class Person {
public $name;
public $age;
public $gender;
function __construct($name, $age, $gender) {
$this->name = $name;
$this->age = $age;
$this->gender = $gender;
}
}
$person = new Person("John Doe", 30, "Male");
$properties = get_object_vars($person);
print_r($properties);
?>
Output:
Array ( [name] => John Doe [age] => 30 [gender] => Male )
So, get_object_vars() retrieves the public properties of an object as an associative array.
The get_object_vars() function can only retrieve the public properties of an object. If you want to retrieve both public and protected properties, you can use the ReflectionObject class.
Here’s an example of how to retrieve both public and protected properties using ReflectionObject:
<?php
class Person {
public $name;
protected $age;
public $gender;
function __construct($name, $age, $gender) {
$this->name = $name;
$this->age = $age;
$this->gender = $gender;
}
}
$person = new Person("John Doe", 30, "Male");
$reflection = new ReflectionObject($person);
$properties = $reflection->getProperties();
$data = [];
foreach ($properties as $property) {
$property->setAccessible(true);
$data[$property->getName()] = $property->getValue($person);
}
print_r($data);
?>
The above code will output:
Array ( [name] => John Doe [age] => 30 [gender] => Male )
So, ReflectionObject allows you to retrieve both public and protected properties of an object, and the getProperties() method retrieves the properties of an object as a list of ReflectionProperty objects. Then, the setAccessible() method is used to make the protected property accessible, and finally, the getValue() method is used to retrieve the value of the property.